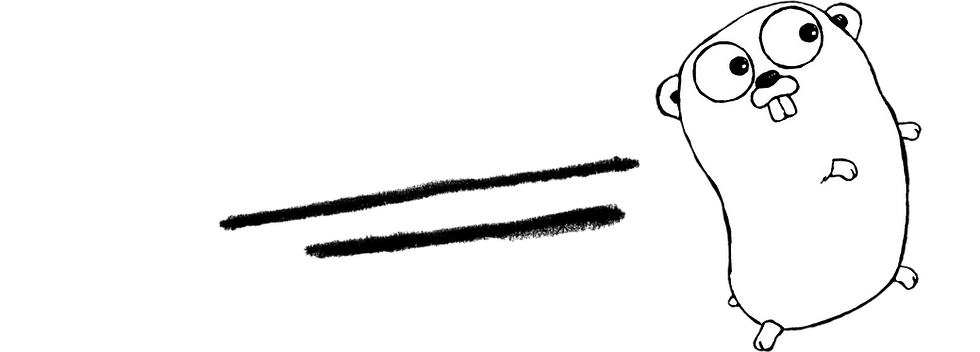
Getting Started with Go Programming
Go, also known as Golang, is an open-source programming language developed by Google in 2007. It is designed to be simple, efficient, and productive, with a focus on concurrency and scalability. In this blog post about getting started with Go Programming, we will guide you through the basics of Go programming and help you get started with it.
Installing Go
The first step to getting started with Go is to install it on your machine. You can download the latest stable version of Go from the official website https://golang.org/dl/. Follow the installation instructions for your operating system.
Your First Go Program
Now that you have installed Go, let's write our first program. Open a text editor and create a new file with the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, world!")
}
Save the file with a .go extension, such as hello.go. Then, open a terminal and navigate to the directory where you saved the file. Type the following command to run the program:
go run hello.go
You should see the message "Hello, world!" printed on the console. Congratulations, you have written and executed your first Go program!
Go Syntax and Basic Concepts
Let's dive a little deeper into Go syntax and some basic concepts.
Variables and Constants
In Go, you declare variables using the var keyword, followed by the variable name and its type. For example:
var x int = 10
You can also declare a variable without specifying its type. Go will infer the type from the value assigned to it:
y := "Hello"
Constants, on the other hand, are declared using the const keyword:
const pi = 3.14159
Functions
Functions in Go are declared using the func keyword. The main function is the entry point for a Go program. For example:
package main
import "fmt"
func add(x int, y int) int {
return x + y
}
func main() {
sum := add(5, 7)
fmt.Println(sum)
}
This program declares a function add that takes two integer arguments and returns their sum. It then calls the add function with arguments 5 and 7 and prints the result to the console.
Control Structures
Go supports the usual control structures such as if/else statements, for loops, and switch statements. Here's an example:
package main
import "fmt"
func main() {
x := 10
if x > 5 {
fmt.Println("x is greater than 5")
} else {
fmt.Println("x is less than or equal to 5")
}
for i := 0; i < 5; i++ {
fmt.Println(i)
}
switch x {
case 10:
fmt.Println("x is 10")
case 20:
fmt.Println("x is 20")
default:
fmt.Println("x is neither 10 nor 20")
}
}
Conclusion
This blog post covered the basics of Go programming, including installation, writing your first program, syntax, and some basic concepts. Go is a powerful and efficient programming language that can be used for a variety of applications. We hope this post has given you a good starting point for learning more about Go and exploring its many features and capabilities.
Remember, practice makes perfect, so keep coding and experimenting with Go to further enhance your skills. Happy coding!
Comentarios