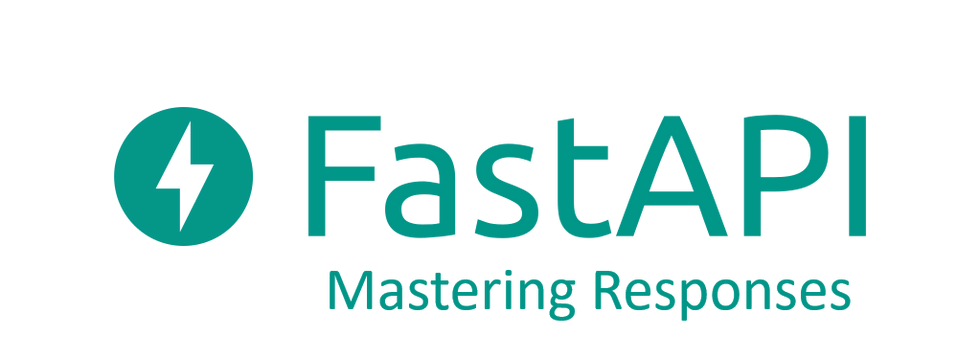
The FastAPI Fundamentals Series
Part-01: Introduction to FastAPI
Part-02: Getting User Input
Part-03: Mastering Responses
Introduction
Welcome back to the FastAPI Fundamentals series! In the previous posts, we explored the basics of FastAPI, building powerful APIs, and handling user input. Now, let's dive deeply into another crucial aspect: FastAPI Responses. Buckle up as we unravel the features that make crafting dynamic and interactive API responses a breeze.
In FastAPI, a response is what your API returns to the client. It can be as simple as a string or as complex as a JSON object or HTML page. FastAPI provides a set of tools to make working with responses easy and efficient.
JSONResponse
By default, FastAPI will return the responses using JSONResponse.
Let's start with the basics. Update your main.py file:
Note: Check the Part-01 of this series to set up your dev environment.
You can either visit http://127.0.0.1:8000/docs/ and test API or usr CURL to test it on the terminal as follows:
$ curl -v http://127.0.0.1:8000/basic_response/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /basic_response/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 04:45:47 GMT
< server: uvicorn
< content-length: 29
< content-type: application/json
<
{"message":"Hello, FastAPI!"}
The response headers confirm that the response is of the JSON type.
Response Models
FastAPI allows you to use Pydantic models to define the structure of your responses. Update your main.py:
Let's test it using CURL:
$ curl --data '{"name":"PraHari Tech", "description":"A Tech Blog"}' -H "Content-Type: application/json" -v http://127.0.0.1:8000/response_model/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> POST /response_model/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
> Content-Type: application/json
> Content-Length: 52
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 05:03:53 GMT
< server: uvicorn
< content-length: 51
< content-type: application/json
<
{"name":"PraHari Tech","description":"A Tech Blog"}
Status Codes
You can specify custom status codes for your responses. Update your main.py:
Now test using http://127.0.0.1:8000/docs or CURL:
$ curl -v http://127.0.0.1:8000/default_status_code/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /default_status_code/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 05:09:49 GMT
< server: uvicorn
< content-length: 42
< content-type: application/json
<
{"message":"Default Status Code Response"}
$ curl -v http://127.0.0.1:8000/status_404/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /status_404/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 404 Not Found
< date: Mon, 11 Dec 2023 05:09:59 GMT
< server: uvicorn
< content-length: 45
< content-type: application/json
<
{"message":"Custom 404 Status Code Response"}
Response Headers
You can include custom headers in your responses and set cookies.
Update your main.py:
Now test using http://127.0.0.1:8000/docs or CURL:
$ curl -v http://127.0.0.1:8000/custom_headers/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /custom_headers/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 05:20:54 GMT
< server: uvicorn
< content-length: 25
< content-type: application/json
< x-my-custom-header: Custom Header Value
<
{"message":"Hello World"}
$ curl -v http://127.0.0.1:8000/set_cookie/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /set_cookie/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 05:21:09 GMT
< server: uvicorn
< content-length: 33
< content-type: application/json
< set-cookie: test_cookie=cookie_value; Path=/; SameSite=lax
<
{"message":"Set Cookie Response"}
Redirects
FastAPI makes it easy to handle redirects. Update your main.py:
Visit http://127.0.0.1:8000/redirect/ to be redirected to the PraHari homepage.
$ curl -v http://127.0.0.1:8000/redirect/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /redirect/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 307 Temporary Redirect
< date: Mon, 11 Dec 2023 06:35:14 GMT
< server: uvicorn
< content-length: 0
< location: https://www.prahari.net/
<
Templates and HTML Responses
FastAPI supports Jinja2 templates for HTML responses. Update your main.py:
Place the below HTML content in a file named index.html within a folder called templates in the same directory as your FastAPI application. This setup is necessary for FastAPI to locate and use the Jinja2 template.
This template includes a basic HTML structure with a title, a heading (<h1>), and a paragraph (<p>). The {{ message }} part is a placeholder that will replace the actual message when FastAPI renders the template.
Visit http://127.0.0.1:8000/html_response/ to see the HTML response.
$ curl -v http://127.0.0.1:8000/html_response/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /html_response/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 06:40:13 GMT
< server: uvicorn
< content-length: 327
< content-type: text/html; charset=utf-8
<
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>FastAPI HTML Response</title>
</head>
<body>
<h1>HTML Response</h1>
<p>This is a simple HTML response generated by FastAPI using Jinja2 templates.</p>
</body>
</html>
Streaming Responses
FastAPI allows you to stream large responses efficiently. Update your main.py:
Visit http://127.0.0.1:8000/stream_response/ to see the streamed response.
$ curl -v http://127.0.0.1:8000/stream_response/
* Trying 127.0.0.1:8000...
* Connected to 127.0.0.1 (127.0.0.1) port 8000
> GET /stream_response/ HTTP/1.1
> Host: 127.0.0.1:8000
> User-Agent: curl/8.4.0
> Accept: /
>
< HTTP/1.1 200 OK
< date: Mon, 11 Dec 2023 06:46:58 GMT
< server: uvicorn
< content-type: application/octet-stream
< Transfer-Encoding: chunked
<
Streamed Response
Conclusion
Congratulations! You've now mastered the art of crafting dynamic and interactive API responses with FastAPI. Whether it's customizing status codes, setting headers, or streaming large responses, FastAPI provides the tools to make your APIs robust and user-friendly.
In the next part of the FastAPI Fundamentals series, we'll explore advanced topics such as dependency injection and security features. Stay tuned for more FastAPI awesomeness!
Happy coding!
תגובות